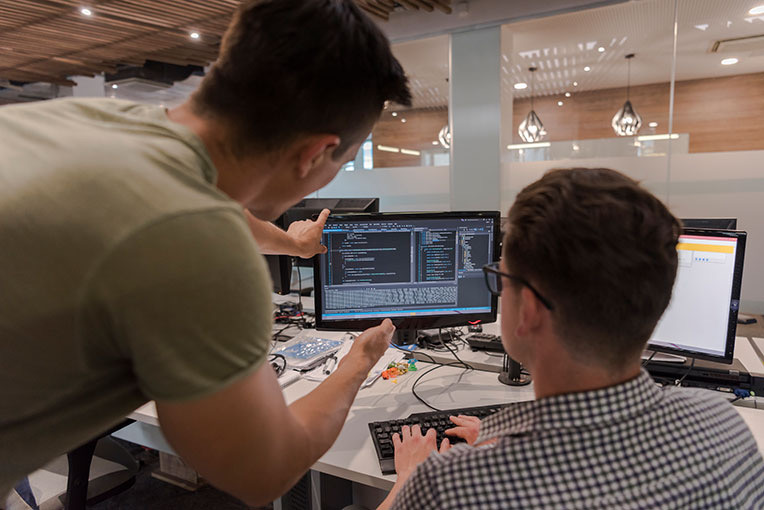
HTML to PDF Converter in C#
Originally posted on https://ironpdf.com/tutorials/html-to-pdf/
Originally posted on YouTube by Iron Software
How to Convert HTML to PDF in ASP.NET C#
Follow these steps:
- Setup C# HTML to PDF .Net Library with Visual Studio
- Create a PDF in Asp.Net C# using a HTML string
- Export online HTML URL to PDF document in C#
- Generate PDF from and existing HTML file
- HTML to PDF Settings, Templates, and Extras
Creating PDF files programmatically in .Net can be a frustrating task. The PDF document file format was designed more for printers than for developers.
In this C# PDF tutorial we will show that with C# we can create PDF documents using HTML as the ‘content’ for the PDF. This ensures that we, as .net coders, do not need to learn proprietary file formats or new APIs. We can easily output dynamic PDF files from our programs and web-applications.
The tool we will be using in this tutorial is IronPDF, a popular C# PDF generation and editing library. This library has comprehensive PDF editing and generation functionality via HTML to PDF. IronPDF stands out in that it supports .Net Framework and .Net Core on Windows, Linux , Azure and MacOS.
With C# and IronPDF, creating PDF documents can be straightforward. Much of the PDF document design and layout can use existing HTML assets or be delegated to web design staff.
This method of dynamic PDF generation in .Net with HTML5 works equally well in console applications, windows forms applications, WPF, as well as websites and MVC. IronPDF is compatible with any .Net Framework project from Version 4 upwards, .Net Core from version 2 upwards.
Getting Set Up with a C# PDF Library
First we will need to download a popular C# PDF Generator (also equally viable for VB.Net, ASP.Net and F#).
In Visual Studio, right click on your project solution explorer and select “Manage Nuget Packages…”. From there simply search for IronPDF and install the latest version… click ok to any dialog boxes that come up.
This will work in any C# .Net Framework project from Framework 4 and above, or .Net Core 2 and above. It will also work just as well in VB.Net projects.
PM > Install-Package IronPdf
https://www.nuget.org/packages/IronPdf
Alternatively, the IronPDF DLL can be downloaded and manually installed to the project or GAC from http://ironpdf.com/packages/IronPdf.zip
Remember to add this statement to the top of any cs class file using IronPDF:
using IronPdf;
1, Creating a PDF with a HTML String in .NET
C# HTML String to PDF is a very efficient and rewarding way to create a new PDF file in C#.
We can simply use the HtmlToPdf.RenderHtmlAsPdf method to turn any HTML (HTML5) string into a PDF. C# HTML to PDF rendering is undertaken by a fully functional version of the Google Chromium engine, embedded within IronPDF DLL.
// Render any HTML fragment or document to HTML
var Renderer = new IronPdf.HtmlToPdf();
var PDF = Renderer.RenderHtmlAsPdf("Hello IronPdf
");
var OutputPath = "HtmlToPDF.pdf";
PDF.SaveAs(OutputPath);
// This neat trick opens our PDF file so we can see the result in our default PDF viewer
System.Diagnostics.Process.Start(OutputPath);
' Render any HTML fragment or document to HTML
Dim Renderer = New IronPdf.HtmlToPdf()
Dim PDF = Renderer.RenderHtmlAsPdf("Hello IronPdf
")
Dim OutputPath = "HtmlToPDF.pdf"
PDF.SaveAs(OutputPath)
' This neat trick opens our PDF file so we can see the result in our default PDF viewer
System.Diagnostics.Process.Start(OutputPath)
RenderHtmlAsPdf fully supports CSS, Javascript and Images. If these assets are on a hard disk, we may wish to set the second parameter of RenderHtmlAsPdf
BaseUrlPath:
var PDF = Renderer.RenderHtmlAsPdf("
", @"C:MyProjectAssets");
// this will render C:MyProjectAssetsimage1.png
Dim PDF = Renderer.RenderHtmlAsPdf("
", "C:MyProjectAssets")
' this will render C:MyProjectAssetsimage1.png
All referenced CSS stylesheets, images and javascript files will be relative to the BaseUrlPath and can be kept in a neat and logical structure. You may also, of course opt to reference images, stylesheets and assets online, including web-fonts such as Google Fonts and even jQuery.
2, Exporting a PDF using Existing HTML URL
Rendering existing URLS as PDFs with C# is very efficient and intuitive. This also allows teams to split PDF design and back-end PDF rendering work across multiple teams.
Lets render a page from Wikipedia.com in the following example:
// Create a PDF from any existing web page
var Renderer = new IronPdf.HtmlToPdf();
var PDF = Renderer.RenderUrlAsPdf("https://en.wikipedia.org/wiki/Portable_Document_Format");
PDF.SaveAs("wikipedia.pdf");
// This neat trick opens our PDF file so we can see the result
System.Diagnostics.Process.Start("wikipedia.pdf");
' Create a PDF from any existing web page
Dim Renderer = New IronPdf.HtmlToPdf()
Dim PDF = Renderer.RenderUrlAsPdf("https://en.wikipedia.org/wiki/Portable_Document_Format")
PDF.SaveAs("wikipedia.pdf")
' This neat trick opens our PDF file so we can see the result
System.Diagnostics.Process.Start("wikipedia.pdf")
You will notice that hyperlinks and even HTML forms are preserved within the PDF generated by our C# code.
When rendering existing web pages we have some tricks we may wish to apply:
Print and Screen CSS
In modern CSS3 we have css directives for both print and screen. We can instruct IronPDF to render “Print” CSSs which are often simplified or overlooked. By default “Screen” CSS styles will be rendered, which IronPDF users have found most intuitive.
Renderer.PrintOptions.CssMediaType = PdfPrintOptions.PdfCssMediaType.Print;
//or
Renderer.PrintOptions.CssMediaType = PdfPrintOptions.PdfCssMediaType.Screen;
Renderer.PrintOptions.CssMediaType = PdfPrintOptions.PdfCssMediaType.Print
'or
Renderer.PrintOptions.CssMediaType = PdfPrintOptions.PdfCssMediaType.Screen
Javascript
IronPDF supports Javascript, jQuery and even AJAX. We may need to instruct IronPDF to wait for JS or ajax to finish running before rendering a snapshot of our web-page.
Renderer.PrintOptions.EnableJavaScript = true;
Renderer.PrintOptions.RenderDelay = 500; //milliseconds
Renderer.PrintOptions.EnableJavaScript = True
Renderer.PrintOptions.RenderDelay = 500 'milliseconds
We can demonstrate compliance with the Javascript standard by rendering an advanced d3.js Javascript chord chart from a csv dataset like this:
// Create a PDF Chart a live rendered dataset using d3.js and javascript
var Renderer = new HtmlToPdf();
var PDF = Renderer.RenderUrlAsPdf("https://bl.ocks.org/mbostock/4062006");
PDF.SaveAs("chart.pdf");
' Create a PDF Chart a live rendered dataset using d3.js and javascript
Dim Renderer = New HtmlToPdf()
Dim PDF = Renderer.RenderUrlAsPdf("https://bl.ocks.org/mbostock/4062006")
PDF.SaveAs("chart.pdf")
Responsive CSS
Responsive web pages are designed to be viewed in a browser. IronPDF does not open a real browser window within your server’s OS. This can lead to responsive elements rendering at their smallest size.
We recommend using Print css media types to navigate this issue. Print CSS should not normally be responsive.
Renderer.PrintOptions.CssMediaType = PdfPrintOptions.PdfCssMediaType.Print;
Renderer.PrintOptions.CssMediaType = PdfPrintOptions.PdfCssMediaType.Print
3, Generating a PDF from an Existing HTML file
We can also render any HTML file on our hard disk. All relative assets such as CSS, images and js will be rendered as if the file had been opened using the file:// protocol.
// Create a PDF from an existing HTML using C#
var Renderer = new IronPdf.HtmlToPdf();
var PDF = Renderer.RenderHTMLFileAsPdf("Assets/TestInvoice1.html");
var OutputPath = "Invoice.pdf";
PDF.SaveAs(OutputPath);
' Create a PDF from an existing HTML using C#
Dim Renderer = New IronPdf.HtmlToPdf()
Dim PDF = Renderer.RenderHTMLFileAsPdf("Assets/TestInvoice1.html")
Dim OutputPath = "Invoice.pdf"
PDF.SaveAs(OutputPath)
This method has the advantage of allowing the developer the opportunity to test the HTML content in a browser during development. We recommend Chrome as it is the web browser on which IronPDF’s rendering engine is based.
To convert XML to PDF you can use XSLT templating to print your XML content to PDF.
Adding Headers And Footers
Headers and footers can be added to PDFs when they are rendered, or to existing PDF files using IronPDF.
With IronPdf, Headers and footers can contain simple text based content using the SimpleHeaderFooter class – or with images and rich html content using the HtmlHeaderFooter class.
// Create a PDF from an existing HTML
var Renderer = new IronPdf.HtmlToPdf();
Renderer.PrintOptions.MarginTop = 50; //millimeters
Renderer.PrintOptions.MarginBottom = 50;
Renderer.PrintOptions.CssMediaType = PdfPrintOptions.PdfCssMediaType.Print;
Renderer.PrintOptions.Header = new SimpleHeaderFooter()
{
CenterText = "{pdf-title}",
DrawDividerLine = true,
FontSize = 16
};
Renderer.PrintOptions.Footer = new SimpleHeaderFooter()
{
LeftText = "{date} {time}",
RightText = "Page {page} of {total-pages}",
DrawDividerLine = true,
FontSize = 14
};
var PDF = Renderer.RenderHTMLFileAsPdf("Assets/TestInvoice1.html");
var OutputPath = "Invoice.pdf";
PDF.SaveAs(OutputPath);
// This neat trick opens our PDF file so we can see the result
System.Diagnostics.Process.Start(OutputPath);
' Create a PDF from an existing HTML
Dim Renderer = New IronPdf.HtmlToPdf()
Renderer.PrintOptions.MarginTop = 50 'millimeters
Renderer.PrintOptions.MarginBottom = 50
Renderer.PrintOptions.CssMediaType = PdfPrintOptions.PdfCssMediaType.Print
Renderer.PrintOptions.Header = New SimpleHeaderFooter() With {
.CenterText = "{pdf-title}",
.DrawDividerLine = True,
.FontSize = 16
}
Renderer.PrintOptions.Footer = New SimpleHeaderFooter() With {
.LeftText = "{date} {time}",
.RightText = "Page {page} of {total-pages}",
.DrawDividerLine = True,
.FontSize = 14
}
Dim PDF = Renderer.RenderHTMLFileAsPdf("Assets/TestInvoice1.html")
Dim OutputPath = "Invoice.pdf"
PDF.SaveAs(OutputPath)
' This neat trick opens our PDF file so we can see the result
System.Diagnostics.Process.Start(OutputPath)
Renderer.PrintOptions.Footer = new SimpleHeaderFooter()
{
LeftText = "{date} {time}",
RightText = "Page {page} of {total-pages}",
DrawDividerLine = true,
FontSize = 14
};
Renderer.PrintOptions.Footer = New SimpleHeaderFooter() With {
.LeftText = "{date} {time}",
.RightText = "Page {page} of {total-pages}",
.DrawDividerLine = True,
.FontSize = 14
}
HTML Headers and Footers
The HtmlHeaderFooter class allows for rich headers and footers to be generated using HTML5 content which may even include images, stylesheets and hyperlinks.
Renderer.PrintOptions.Footer = new HtmlHeaderFooter() { HtmlFragment = "page {page} of {total-pages}" };
Renderer.PrintOptions.Footer = New HtmlHeaderFooter() With {.HtmlFragment = "page {page} of {total-pages}"}
Dynamic Data in PDF Headers and Footers
We may “mail-merge” content into the text and even HTML of headers and footers using placeholders such as:
- {page} for the current page number
- {total-pages} for the total number of pages in the PDF
- {url} for the URL of the rendered PDF if rendered from a web page
- {date} for today’s date
- {time} for the current time
- {html-title} for the title attribute of the rendered HTML document
- {pdf-title} for the document title, which may be set via the PrintOptions
C# HTML to PDF Settings
There are many nuances to how our users and clients may expect PDF content to be rendered.
The HtmlToPdf class contains a PrintOptions object which can be used to set these options.
For example we may wish to choose to only accept “print” style CSS3 directives:
Renderer.PrintOptions.CssMediaType = PdfPrintOptions.PdfCssMediaType.Print;
Renderer.PrintOptions.CssMediaType = PdfPrintOptions.PdfCssMediaType.Print
We may also wish to change the size of our print margins to create more whitespace on the page, to make room for large headers or footers, or even set zero margins for commercial printing of brochures or posters:
Renderer.PrintOptions.MarginTop = 50; //millimeters
Renderer.PrintOptions.MarginBottom = 50;
Renderer.PrintOptions.MarginTop = 50 'millimeters
Renderer.PrintOptions.MarginBottom = 50
We may wish to turn on or off background images from html elements:
Renderer.PrintOptions.PrintHtmlBackgrounds = true;
Renderer.PrintOptions.PrintHtmlBackgrounds = True
It is also possible to set our output PDFs to be rendered on any virtual paper size – including portrait and landscape sizes and even custom sizes which may be set in millimeters or inches.
Renderer.PrintOptions.PaperSize = PdfPrintOptions.PdfPaperSize.A4;
Renderer.PrintOptions.PaperOrientation = PdfPrintOptions.PdfPaperOrientation.Landscape;
Renderer.PrintOptions.PaperSize = PdfPrintOptions.PdfPaperSize.A4
Renderer.PrintOptions.PaperOrientation = PdfPrintOptions.PdfPaperOrientation.Landscape
Full documentation of C# Html to PDF Settings may be found at https://ironpdf.com/c%23-pdf-documentation/html/T_IronPdf_PdfPrintOptions.htm
The full set of PDF PrintOptions includes:
- CreatePdfFormsFromHtml Turns all HTML forms elements into editable PDF forms.
- CssMediaType Enables Media=”screen” or “print” CSS Styles and StyleSheets.
- CustomCssUrl Allows a custom CSS style-sheet to be applied to Html before rendering. May be a local file path, or a remote url.
- DPI Printing output DPI. 300 is standard for most print jobs. Higher resolutions produce clearer images and text, but also larger PDF files.
- EnableJavaScript Enables JavaScript and Json to be executed before the page is rendered. Ideal for printing from Ajax / Angular Applications. Also see RenderDelay.
- FirstPageNumber First page number to be used in PDF headers and footers.
- FitToPaperWidth Where possible, fits the PDF content to 1 page width.
- Footer Sets the header content for every PDF page as Html or a String. Supports ‘mail-merge’
- GrayScale Outputs a black-and-white PDF
- Header Sets the footer content for every PDF page as Html or String. Supports ‘mail-merge’
- InputEncoding The input character encoding as a string
- JpegQuality Quality of any image that must be re-sampled. 0-100
- MarginBottom Paper margin in millimeters. Set to zero for border-less and commercial printing applications
- MarginLeft Paper margin in millimeters
- MarginRight Paper margin in millimeters
- MarginTop Paper margin in millimeters. Set to zero for border-less and commercial printing applications
- PaperOrientation The PDF paper orientation.
- PaperSize Set an output paper size for PDF pages. System.Drawing.Printing.PaperKind. Use SetCustomPaperSize(int width, int height) for custom sizes
- PrintHtmlBackgrounds Prints background-colors and images from Html
- RenderDelay Milliseconds delay to wait after Html is rendered before printing. This can use useful when considering the rendering of JavaScript, Ajax or animations
- Title PDF Document Name and Title meta-data. Not required
- Zoom The zoom level in %. Enlarges the rendering size of Html documents
HTML Templating
To template or “batch create” PDFs is a common requirement for Intranet and website developers.
Rather than templating a PDF document itself, with IronPDF we can template our HTML using existing, well tried technologies. When the HTML template is combined with data from a query-string or database we end up with a dynamically generated PDF document.
In the simplest instance, using the C# String.Format method is effective for basic “mail-merge”
String.Format("Hello {0} !","World");
String.Format("Hello {0} !","World")
If the Html file is longer, often we can use arbitrary placeholders such as [[NAME]]
and replace them with real data later.
The following example will create 3 PDFs, each personalized to a user.
var HtmlTemplate = "[[NAME]]
";
var Names = new[] { "John", "James", "Jenny" };
foreach (var name in Names) {
var HtmlInstance = HtmlTemplate.Replace("[[NAME]]", name);
var Pdf = Renderer.RenderHtmlAsPdf(HtmlInstance);
Pdf.SaveAs(name + ".pdf");
}
Dim HtmlTemplate = "[[NAME]]
"
Dim Names = { "John", "James", "Jenny" }
For Each name In Names
Dim HtmlInstance = HtmlTemplate.Replace("[[NAME]]", name)
Dim Pdf = Renderer.RenderHtmlAsPdf(HtmlInstance)
Pdf.SaveAs(name & ".pdf")
Next name
Advanced Templating With Handlebars.Net
A sophisticated method to merge C# data with HTML for PDF generation is using the Handlebars Templating standard.
Handlebars makes it possible to create dynamic html from C# objects and class instances including database records. Handlebars is particularly effective where a query may return an unknown number of rows such as in the generation of an invoice.
We must first add an additional Nuget Package to our project: https://www.nuget.org/packages/Handlebars.Net/
string source =
@"
{{title}}
{{body}}
";
var template = Handlebars.Compile(source);
var data = new {
title = "My new post",
body = "This is my first post!"
};
var result = template(data);
/* Would render:
My New Post
This is my first post!
*/
Dim source As String = "
{{title}}
{{body}}
"
Dim template = Handlebars.Compile(source)
Dim data = New With {
Key .title = "My new post",
Key .body = "This is my first post!"
}
Dim result = template(data)
' Would render:
'
' My New Post
'
' This is my first post!
'
'
'
VB C#
To render this html we can simply use the RenderHtmlAsPdf method.
IronPdf.HtmlToPdf Renderer = new IronPdf.HtmlToPdf();
Renderer.RenderHtmlAsPdf(HtmlInstance).SaveAs("Handelbars.pdf")
Dim Renderer As New IronPdf.HtmlToPdf()
Renderer.RenderHtmlAsPdf(HtmlInstance).SaveAs("Handelbars.pdf")
You can learn more about the handlebars html templating standard and its C# using from https://github.com/rexm/Handlebars.Net
Page Breaks using HTML5
A common requirement in a PDF document is for pagination. Developers need to control where PDF pages start and end for a clean, readable layout.
The easiest way to do this is with a less known CSS trick which will render a page break into any printed HTML document.
The provided HTML works, but is hardly best practice. We found this example to be very helpful in our understanding of a neat and tidy way to lay out multi-page html content.
Paginated HTML
This is Page 1
This is Page 2
This is Page 3
The FAQ outlines more tips and tricks with Page Breaks
Attaching a Cover Page to a PDF
IronPDF makes it easy to Merge pdf documents. The most common usage of this technique is to add a cover page or back page to an existing rendered PDF document.
To do so we first render a cover page, and then use the PdfDocument.Merge
static method to combine the 2 documents.
var PDF = Renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf/");
PdfDocument.Merge(new PdfDocument("CoverPage.pdf"), PDF).SaveAs("Combined.Pdf");
Dim PDF = Renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf/")
PdfDocument.Merge(New PdfDocument("CoverPage.pdf"), PDF).SaveAs("Combined.Pdf")
Adding a WaterMark
A final C# PDF trick is to add a watermark to PDF documents. This can be used to add a notice to each page that a document is “confidential” or a “sample”.
// Stamps a watermark onto a new or existing PDF
IronPdf.HtmlToPdf Renderer = new IronPdf.HtmlToPdf();
var pdf = Renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf");
pdf.WatermarkAllPages("SAMPLE
", PdfDocument.WaterMarkLocation.MiddleCenter, 50, -45, "https://www.nuget.org/packages/IronPdf");
pdf.SaveAs(@"C:PathToWatermarked.pdf");
' Stamps a watermark onto a new or existing PDF
Dim Renderer As New IronPdf.HtmlToPdf()
Dim pdf = Renderer.RenderUrlAsPdf("https://www.nuget.org/packages/IronPdf")
pdf.WatermarkAllPages("SAMPLE
", PdfDocument.WaterMarkLocation.MiddleCenter, 50, -45, "https://www.nuget.org/packages/IronPdf")
pdf.SaveAs("C:PathToWatermarked.pdf")
Downloading this Tutorial as C# Source Code
The full free Html to PDF C# Source Code for this tutorial is available to download as a zipped Visual Studio 2017 project file.
Download this tutorial as a Visual Studio project
The free download contains working C# PDF code examples code for:
- Html Strings to PDFs using C#
- Html files (supporting CSS, Javascript and images) to PDF
- C# HTML to PDF using a URL
- C# PDF editing and settings examples
- Rendering Javascript canvas charts such as d3.js to a PDF
- The PDF Library for C#
Comparison with Other PDF Libraries
PDFSharp
PDFSharp is a free open source library which allows logical editing and creation of Pdf documents in .Net.
A key difference between PDFSharp and IronPDF is that IronPDF has an embedded Web Browser which allows faithful creation of PDFs from HTML, CSS, JS and images.
The IronPDF API also differs from PDFSharp in that it is based around use cases rather than the technical structure of PDF documents. Many find this more logical and intuitive to use.
WKHtmlToPdf
WKHtmlToPdf is a free, open source library written in C++ which allows PDF documents to be rendered from HTML.
A key difference between WKHtmlToPdf and IronPDF is that IronPDF is written in C# and is stable and thread safe for use in .NET applications and Websites.
The IronPDF API also differs from WKHtmlToPdf in that it has a large and advanced API allowing PDF documents to be edited, Manipulated Imported, Exported, Signed, Secured and Watermarked.
iTextSharp
iTextSharp is an open source partial port of the iText java library for PDF generation and editing.
A key difference between iTextSharp and IronPDF is that IronPDF is has more advanced and accurate HTML-To-PDF rendering by using an embedded Chrome based web browser.
The IronPDF API also differs from iTextSharp in that IronPDF has explicit licenses for commercial or private usage, where as iTextSharp’s AGLP license is only suitable for applications where the full source code is presented for free to every user – even users across the internet.
A full breakdown of the differences is available in our iTextSharp FAQ.
Other Commercial Libraries
Aspose PDF, Spire PDF, EO PDF, and SelectPdf are competitor .Net commercial PDF libraries by other vendors. It is unfair for tutorials on this website to comment on them comparatively, as we clearly believe in the quality of IronPDF. Suffice to say: we believe IronPDF to have a comparatively strong feature set, excellent .Net Core compatibility and a fair price point.
Downloadable C# PDF QuickStart Guide
To make developing PDFs in your .Net applications easier, we have compiled a quick-start guide as a PDF document. This “Cheat-Sheet” provide quick access to common functions and examples for generating and editing PDFs in C# and VB.Net – and may help save time in getting started using IronPDF in your .Net project.
Explore this Tutorial on GitHub
The source code for this Html-To-Pdf project is available in C# and VB.NET on GitHub.
Browsing the source code may provide insights into how to get more out of Iron PDF and also provide an easy way to get up and running in just a few minutes.
The projects are saves as Microsoft Visual Studio 2017 projects but the code is compatible with any .Net IDE.
- C# HTML to PDF Code Project on Github
- VB.NET HTML to PDF Code Project on Github
Going Forwards
Developers may also be interested in the IronPdf.PdfDocument Class reference: https://ironpdf.com/c%23-pdf-documentation/html/T_IronPdf_PdfDocument.htm
This object model shows how PDF documents may be:
- Encrypted and password protected
- Edited or ‘stamped’ with new html content
- Enhanced with foreground and background images
- Merged, joined, truncated and spliced at a page or document level
- OCR processed to extract plain text and images
No Comments
Sorry, the comment form is closed at this time.