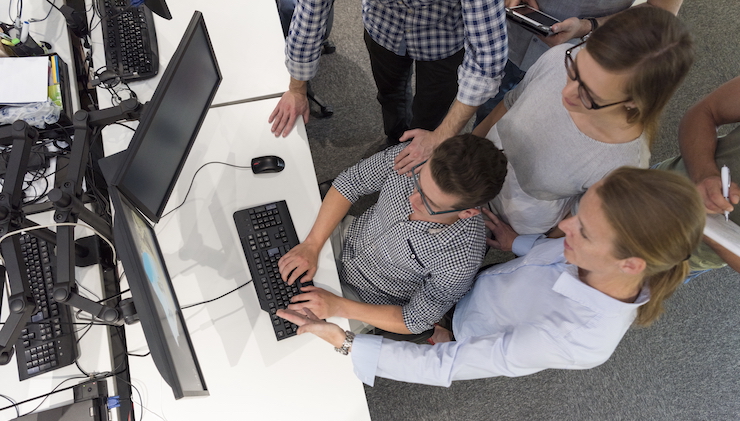
Use C# to Open & Write an Excel File in .Net
Originally posted on https://ironsoftware.com/csharp/excel/tutorials/csharp-open-write-excel-file/
How to open an Excel file in C#
- Install IronXL Excel Library from NuGet or the DLL download
- Use the WorkBook.Load method to read any XLS, XLSX or CSV document.
- Get Cell values using intuitive syntax:
sheet["A11"].DecimalValue
Install the Excel Library to your Visual Studio Project
IronXL.Excel provides a versatile, advanced, and efficient library for opening, reading, editing and saving Excel files in .NET.
The first step will be to install IronXL.Excel, and this is most easily achieved using our NuGet package, although you may also choose to manually install the DLL to your project or to your global assembly cache.
PM > Install-Package IronXL.Excel
Open an Excel File
using IronXL;
using System;
WorkBook workbook = WorkBook.Load("test.xlsx");
WorkSheet sheet = workbook.DefaultWorkSheet;
Range range = sheet["A2:A8"];
decimal total = 0;
//iterate over range of cells
foreach (var cell in range)
{
Console.WriteLine("Cell {0} has value '{1}'", cell.RowIndex, cell.Value);
if (cell.IsNumeric)
{
//Get decimal value to avoid floating numbers precision issue
total += cell.DecimalValue;
}
}
//check formula evaluation
if (sheet["A11"].DecimalValue == total)
{
Console.WriteLine("Basic Test Passed");
}
Write and Save Changes to the Excel File
sheet["B1"].Value = 11.54;
//Save Changes
workbook.SaveAs("test.xlsx");
Summary
In summary, IronXL.Excel is a stand alone .Net software library library for reading a wide range of spreadsheet formats. It does not require Microsoft Excel to be installed, nor depend on Interop.
Further Reading
To learn more about working with IronXL, you may wish to look at the other tutorials within this section, and also the examples on our homepage; which most developers find enough to get them started.
Our Object Reference with specific reference to the WorkBook
class.