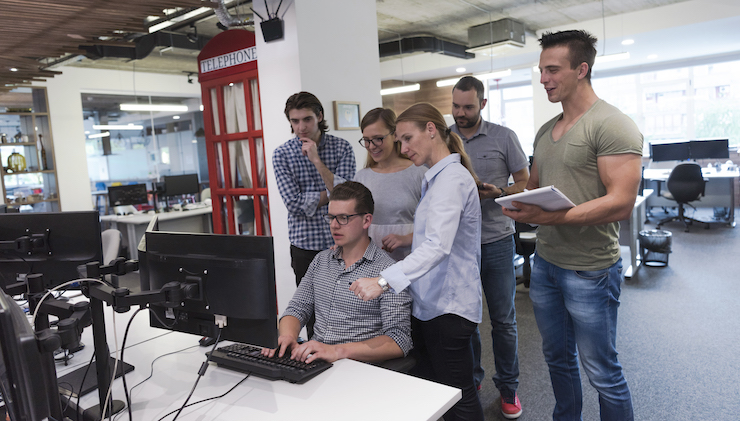
Using C# to Create Excel Files in .Net
Originally posted on https://ironsoftware.com/csharp/excel/tutorials/create-excel-file-net/
Creating Excel files in C# can be simple even without dependency on the legacy Microsoft.Office.Interop.Excel library.
In this tutorial we will use IronXL to create an Excel WorkBook file on any platform that supports .Net Framework 4.5 or .Net Core.
Installation
Before we start we need to install the IronXL Excel NuGet Package.
PM > Install-Package IronXL.Excel
https://www.nuget.org/packages/IronXL.Excel/
As an alternative, the IronXL.Dll can be downloaded and added to your project.
Create and save an Excel File
using IronXL;
//default file format is XLSX, we can override it using CreatingOptions
WorkBook workbook = WorkBook.Create(ExcelFileFormat.XLSX);
var sheet = workbook.CreateWorkSheet("example_sheet");
sheet["A1"].Value = "Example";
//set value to multiple cells
sheet["A2:A4"].Value = 5;
sheet["A5"].Style.SetBackgroundColor("#f0f0f0");
//set style to multiple cells
sheet["A5:A6"].Style.Font.Bold = true;
//set formula
sheet["A6"].Value = "=SUM(A2:A4)";
if (sheet["A6"].IntValue == sheet["A2:A4"].IntValue)
{
Console.WriteLine("Basic test passed");
}
workbook.SaveAs("example_workbook.xlsx");
Moving Forward
To learn more about creating Excel files in C# .Net, we encourage you to work with this code sample and explore the object reference to move forwards to meet you own project’s specific need.